Android Retrofit
Retrofit은 HTTP REST API 구현을 위한 라이브러리로 사용됩니다.
서버와 클라이언트간 Http 통신을 위한 인터페이스를 뜻합니다.
쉽게 말해, 클라이언트에서 서버로 어떠한 요청을 보내면 서버는 그 요청에 대한 응답을 클라이언트로 보내주게 되는데, 이 일련의 과정들을 쉽게 사용 할 수 있도록 도와주는 역할을 하는 것이 바로 Retrofit 입니다.
HTTP REST
웹에서 사용하는 Architecture의 한 형식. 네트워크 상에서 클라이언트와 서버 간의 통신 방식을 말합니다.
- HTTP에서는 GET, POST, PUT, DELETED 등의 Method를 제공합니다.
- 클라이언트에 대한 응답은 xml, json, text, rss 등으로 전달하게 됩니다.
Retrofit은 REST API을 구현한 상태입니다.
그래서 간단하게 GET, POST, PUT, DELETED 등을 전달하면 서버에서 처리 후 xml, json, text, rss 등으로 응답을 제공받을 수 있는 형태입니다.
Retrofit 설치
https://square.github.io/retrofit/
gradle에 추가해줍니다.
1
2
3
4
|
implementation 'androidx.recyclerview:recyclerview:1.1.0'
implementation 'androidx.cardview:cardview:1.0.0'
implementation 'com.squareup.retrofit2:retrofit:2.5.0'
implementation 'com.squareup.retrofit2:converter-gson:2.5.0'
|
Create Interface for Retrofit, Retrofitclient
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
object RetrofitClient {
private var retrofit:Retrofit? = null
fun getClient(baseUrl:String?):Retrofit{
if(retrofit == null)
{
retrofit = Retrofit.Builder()
.baseUrl(baseUrl)
.addConverterFactory(GsonConverterFactory.create())
.build()
}
return retrofit!!
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
인터페이스의 Get url은 newsapi.org페이지 내에서 Document로 가시면 확인가능합니다.
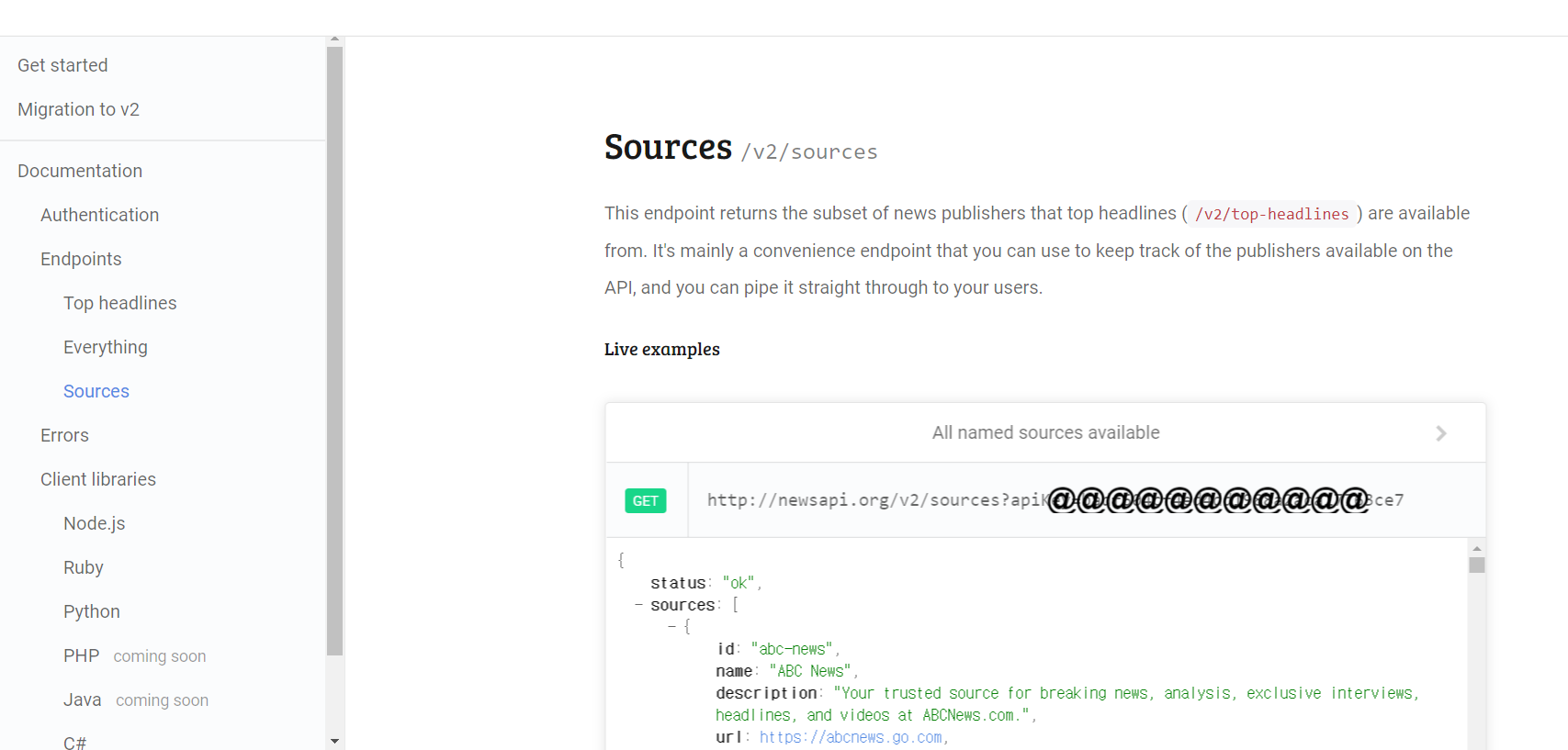
보통 Get주소는 뒤에 본인 apikey를 작성하시면 됩니다. base url을 이미 설정한 경우 v2/~부터 작성하시면 됩니다.
위 url을 통해 json 파일을 살펴보면 source 파일에는 id, name, description 등등의 키가 있는 걸 확인할 수 있습니다.
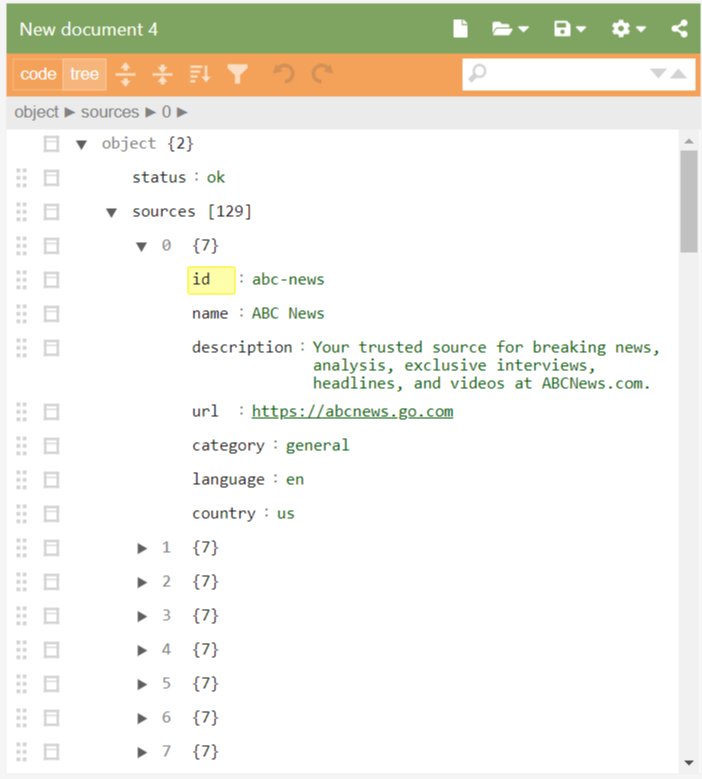
저는 sources 파일의 키값을 하나씩 받는 model을 아래와 같이 작성해주었습니다.
1
2
3
4
5
6
7
8
9
10
11
12
|
package com.example.myapplication.Model
class Source {
var id:String?=null
var name:String?=null
var description:String?=null
var url:String?=null
var category:String?=null
var language:String?=null
var country:String?=null
//json파일에서 필요한 정보
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
cs |
위에 소스를 인터페이스에서 받아오기 위해 일단 웹사이트 모델을 하나 더 만들어줬습니다.
1
2
3
4
5
6
7
8
9
|
class WebSite {
var status: String? = null
var sources:List<Source>?=nullclass WebSite {
var status: String? = null
var sources:List<Source>?=null
//위에 Source는 모델 클래스, Source 클래스를 이용해 n번째 데이터까지 담아서 어댑터에서 넘겨줌
constructor()
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
cs |
이제 웹사이트를 인터페이스에서 불러옵니다.
1
2
3
4
5
|
interface NewsService {
@get:GET("v2/sources?apiKey=key값")
val sources: Call<WebSite>
//website는 모델 클래스
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
이제 이를 저장할 어댑터를 만들어 데이터를 관리해줍니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
class ListSourceAdapter (private val context: Context, private val webSite: WebSite):RecyclerView.Adapter<LIstSourceViewHolder>()
{
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): LIstSourceViewHolder {
val itemView = inflater.inflate(R.layout.source_news_layout, parent, false)
return LIstSourceViewHolder(itemView)
}
override fun getItemCount(): Int {
return webSite.sources!!.size
}
override fun onBindViewHolder(holder: LIstSourceViewHolder, position: Int) {
// holder에 모델 source의 key가 name인 value 값을 넣어줌
holder.setItemClickListener(object:ItemClickListener
{
override fun onClick(view: View, position: Int) {
Toast.makeText(context, "Will be implement in next step", Toast.LENGTH_SHORT).show()
}
})
}
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
뉴스의 헤드라인도 위와 동일하게 받으면 됩니다. 헤드라인은 아래 코드로 받아줄 수 있습니다.
1
2
3
4
5
6
7
|
package com.example.myapplication.Model
class News {
var status:String?=null
var totalResult:Int=0
var articles:MutableList<Article>?=null
}
http://colorscripter.com/info#e" target="_blank" style="color:#e5e5e5text-decoration:none">Colored by Color Scripter
|
cs |
참고링크
https://thdev.tech/androiddev/2016/11/13/Android-Retrofit-Intro/
Android HTTP 통신을 위한 Retrofit 사용하기 |
I’m an Android Developer.
thdev.tech
'Android Studio' 카테고리의 다른 글
[SQL]공부하기(SQLite, SQL 문) (0) | 2020.04.23 |
---|---|
[Android Studio] 코틀린으로 뉴스 리더 어플 만들기2 (0) | 2020.03.22 |
[Android Studio] CreateProcess error=2 해결 (0) | 2020.03.02 |
[Android Studio] Adapter 사용하기 (0) | 2020.02.28 |
[Android Studio] startActivityForResult 사용해보기 (0) | 2020.02.27 |